By: Team CS2113 T13-2
Since: Aug 2018
Licence: MIT
- 1. Setting up
- 2. Design
- 3. Implementation
- 4. Documentation
- 5. Testing
- 6. Dev Ops
- Appendix A: Product Scope
- Appendix B: User Stories
- Appendix C: Use Cases
- Appendix D: Non Functional Requirements
- Appendix E: Glossary
- Appendix F: Instructions for Manual Testing
- F.1. Launch and Shutdown
- F.2. Registering and logging in
- F.3. Announcing new announcement
- F.4. Checking new announcement
- F.5. Blocking user from posting
- F.6. Setting a user as admin or setting an admin as user
- F.7. Creating a module
- F.8. Creating a thread
- F.9. Creating a comment
- F.10. Updating a thread title
- F.11. Updating a comment content
- F.12. Deleting a thread
- F.13. Deleting a comment
- F.14. Listing all modules
- F.15. Selecting a module to list all threads
- F.16. Selecting a thread to list all comments
- F.17. Updating a module
- F.18. Deleting a module
- F.19. Updating password
- F.20. Deleting a user(Admin)
- F.21. Deleting a user(User)
1. Setting up
1.1. Prerequisites
-
JDK
9
or laterJDK 10
on Windows will fail to run tests in headless mode due to a JavaFX bug. Windows developers are highly recommended to use JDK9
. -
IntelliJ IDE
IntelliJ by default has Gradle and JavaFx plugins installed.
Do not disable them. If you have disabled them, go toFile
>Settings
>Plugins
to re-enable them.
1.2. Setting up the project in your computer
-
Fork this repo, and clone the fork to your computer
-
Open IntelliJ (if you are not in the welcome screen, click
File
>Close Project
to close the existing project dialog first) -
Set up the correct JDK version for Gradle
-
Click
Configure
>Project Defaults
>Project Structure
-
Click
New…
and find the directory of the JDK
-
-
Click
Import Project
-
Locate the
build.gradle
file and select it. ClickOK
-
Click
Open as Project
-
Click
OK
to accept the default settings -
Open a console and run the command
gradlew processResources
(Mac/Linux:./gradlew processResources
). It should finish with theBUILD SUCCESSFUL
message.
This will generate all resources required by the application and tests.
1.3. Verifying the setup
-
Run the
MainApp
and try a few commands -
Run the tests to ensure they all pass.
1.4. Configurations to do before writing code
1.4.1. Configuring the coding style
This project follows oss-generic coding standards. IntelliJ’s default style is mostly compliant with ours but it uses a different import order from ours. To rectify,
-
Go to
File
>Settings…
(Windows/Linux), orIntelliJ IDEA
>Preferences…
(macOS) -
Select
Editor
>Code Style
>Java
-
Click on the
Imports
tab to set the order-
For
Class count to use import with '*'
andNames count to use static import with '*'
: Set to999
to prevent IntelliJ from contracting the import statements -
For
Import Layout
: The order isimport static all other imports
,import java.*
,import javax.*
,import org.*
,import com.*
,import all other imports
. Add a<blank line>
between eachimport
-
Optionally, you can follow the UsingCheckstyle.adoc document to configure Intellij to check style-compliance as you write code.
1.4.2. Updating documentation to match your fork
After forking the repo, the documentation will still have the SE-EDU branding and refer to the se-edu/addressbook-level4
repo.
If you plan to develop this fork as a separate product (i.e. instead of contributing to se-edu/addressbook-level4
), you should do the following:
-
Configure the site-wide documentation settings in
build.gradle
, such as thesite-name
, to suit your own project. -
Replace the URL in the attribute
repoURL
inDeveloperGuide.adoc
andUserGuide.adoc
with the URL of your fork.
1.4.3. Setting up CI
Set up Travis to perform Continuous Integration (CI) for your fork. See UsingTravis.adoc to learn how to set it up.
After setting up Travis, you can optionally set up coverage reporting for your team fork (see UsingCoveralls.adoc).
Coverage reporting could be useful for a team repository that hosts the final version but it is not that useful for your personal fork. |
Optionally, you can set up AppVeyor as a second CI (see UsingAppVeyor.adoc).
Having both Travis and AppVeyor ensures your App works on both Unix-based platforms and Windows-based platforms (Travis is Unix-based and AppVeyor is Windows-based) |
1.4.4. Getting started with coding
When you are ready to start coding,
-
Get some sense of the overall design by reading Section 2.1, “Architecture”.
-
Take a look at Appendix A, Product Scope.
2. Design
2.1. Architecture
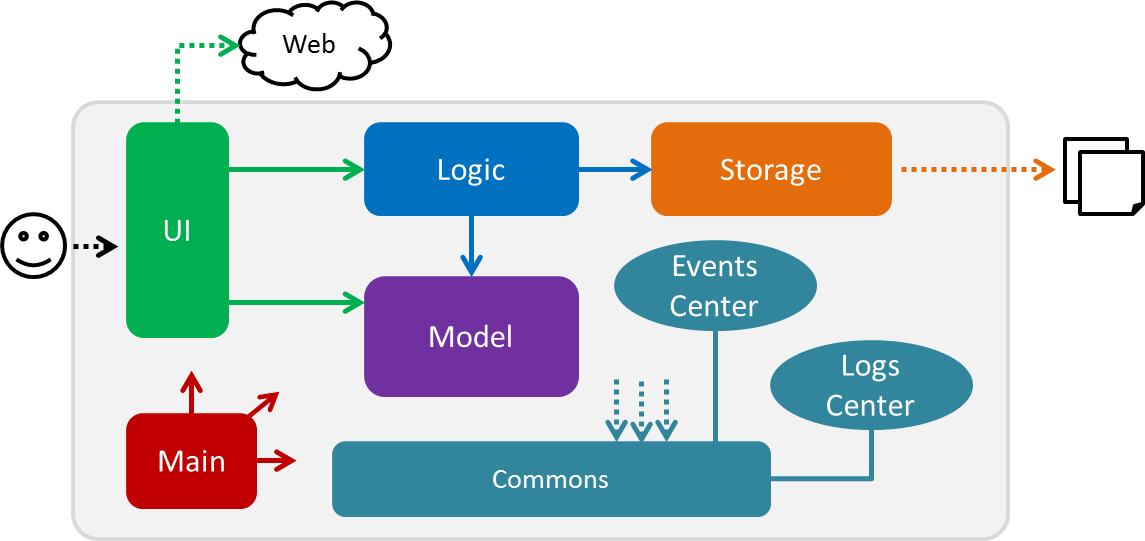
The Architecture Diagram given above explains the high-level design of the App. Given below is a quick overview of each component.
The .pptx files used to create diagrams in this document can be found in the diagrams folder. To update a diagram, modify the diagram in the pptx file, select the objects of the diagram, and choose Save as picture .
|
Main
has only one class called MainApp
. It is responsible for,
-
At app launch: Initializes the components in the correct sequence, and connects them up with each other.
-
At shut down: Shuts down the components and invokes cleanup method where necessary.
Commons
represents a collection of classes used by multiple other components. Two of those classes play important roles at the architecture level.
-
EventsCenter
: This class (written using Google’s Event Bus library) is used by components to communicate with other components using events (i.e. a form of Event Driven design) -
LogsCenter
: Used by many classes to write log messages to the App’s log file.
The rest of the App consists of four components.
Each of the four components
-
Defines its API in an
interface
with the same name as the Component. -
Exposes its functionality using a
{Component Name}Manager
class.
For example, the Logic
component (see the class diagram given below) defines it’s API in the Logic.java
interface and exposes its functionality using the LogicManager.java
class.
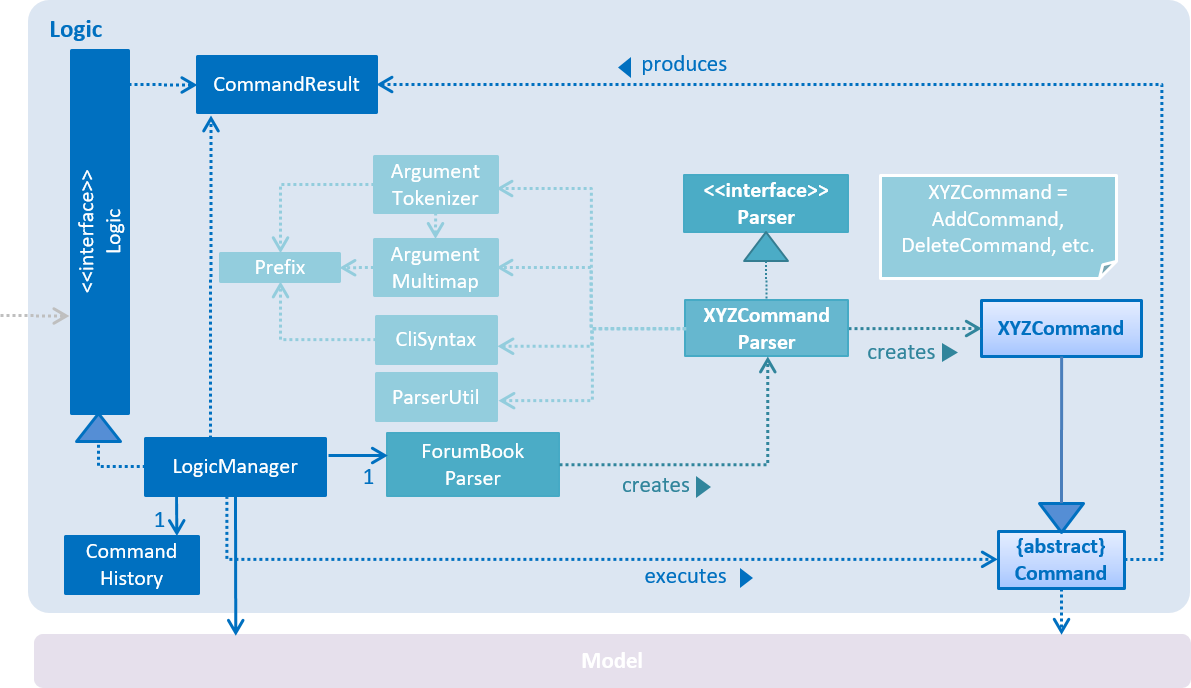
Events-Driven nature of the design
The Sequence Diagram below shows how the components interact for the scenario where the user issues the command deleteModule mCode/CS2113
.
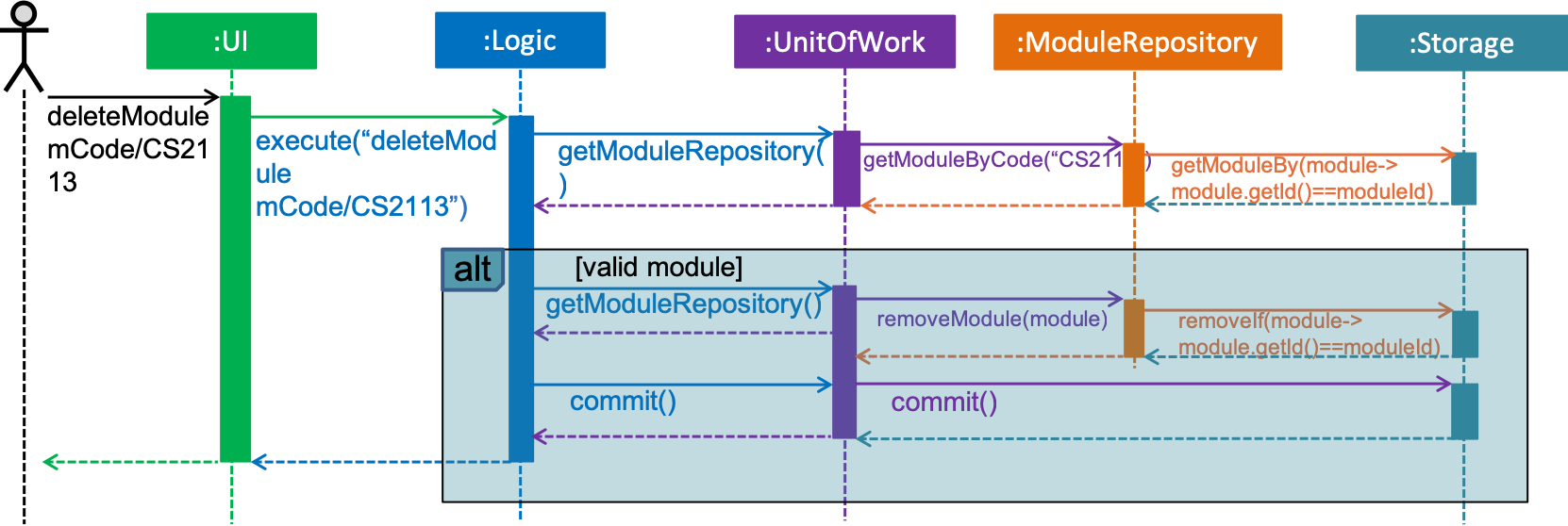
deleteModule mCode/CS2113
command (part 1)
Note how the Model simply raises a ForumBookChangedEvent when the Forum Book data are changed, instead of asking the Storage to save the updates to the hard disk.
|
The diagram below shows how the EventsCenter
reacts to that event, which eventually results in the updates being saved to the hard disk and the status bar of the UI being updated to reflect the 'Last Updated' time.
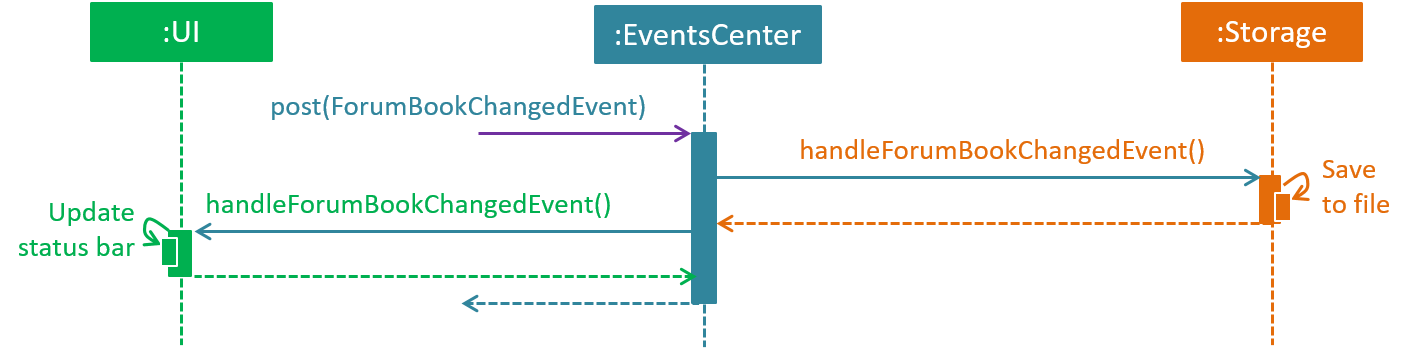
delete m/CS2113 i/1
command (part 2)
Note how the event is propagated through the EventsCenter to the Storage and UI without Model having to be coupled to either of them. This is an example of how this Event Driven approach helps us reduce direct coupling between components.
|
The sections below give more details of each component.
2.2. UI component
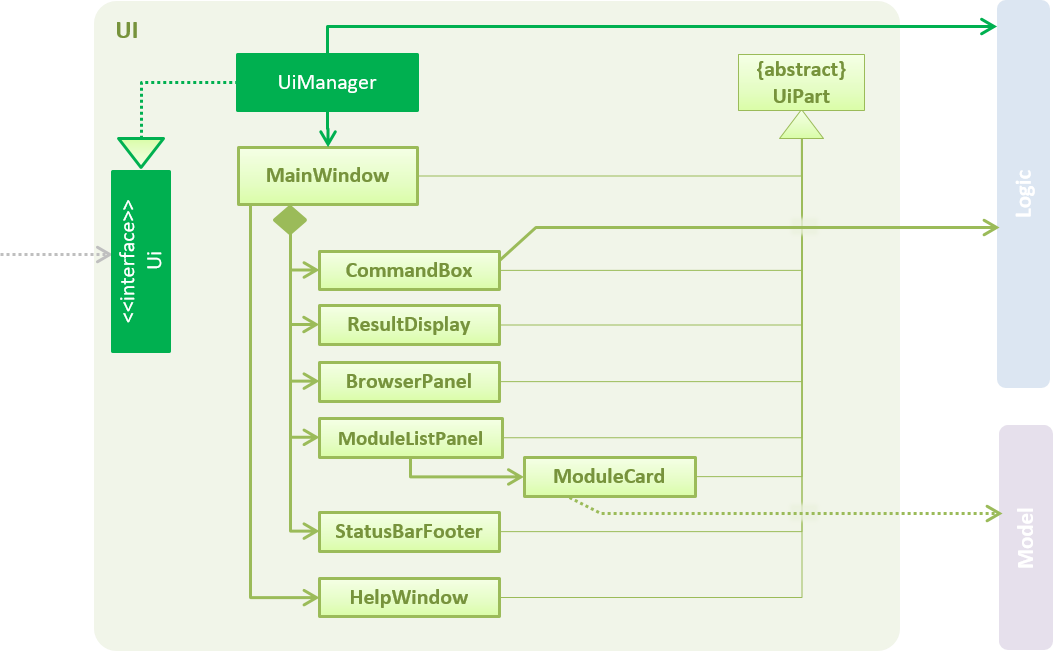
API : Ui.java
The UI consists of a MainWindow
that is made up of parts e.g.CommandBox
, ResultDisplay
etc. All these, including the MainWindow
, inherit from the abstract UiPart
class.
The UI
component uses JavaFx UI framework. The layout of these UI parts are defined in matching .fxml
files that are in the src/main/resources/view
folder. For example, the layout of the MainWindow
is specified in MainWindow.fxml
The UI
component,
-
Executes user commands using the
Logic
component. -
Binds itself to some data in the
Model
so that the UI can auto-update when data in theModel
change. -
Responds to events raised from various parts of the App and updates the UI accordingly.
2.3. Logic component
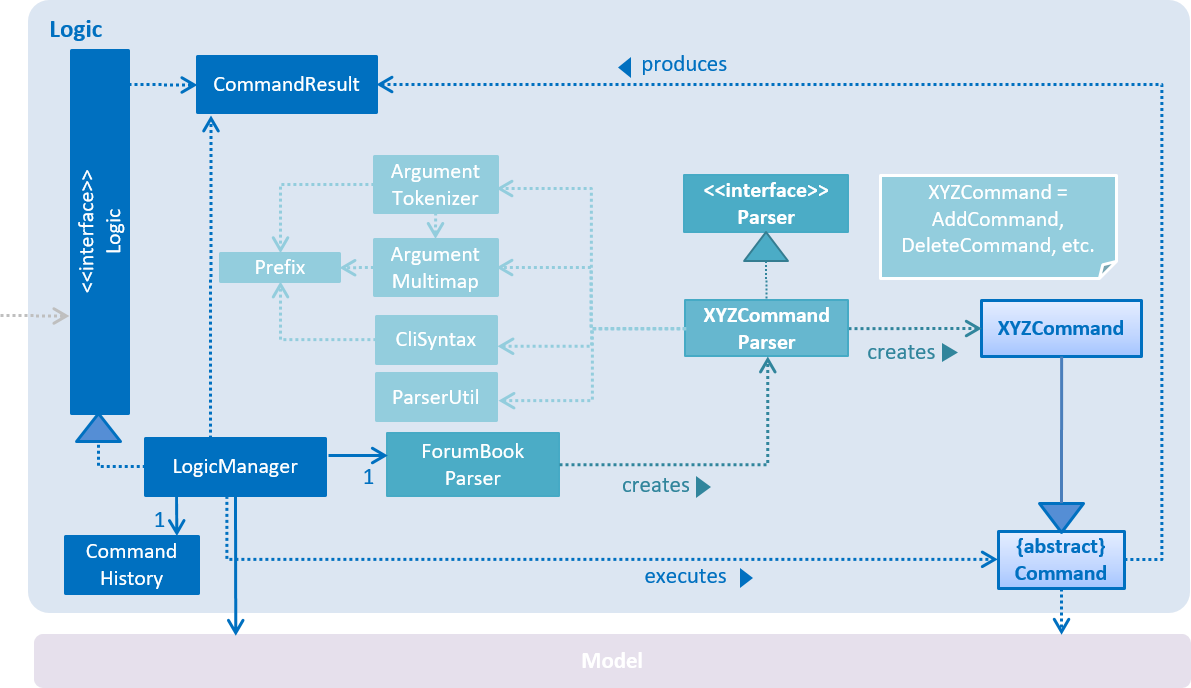
API :
Logic.java
-
Logic
uses theForumBookParser
class to parse the user command. -
This results in a
Command
object which is executed by theLogicManager
. -
The command execution can affect the
Model
(e.g. adding a person) and/or raise events. -
The result of the command execution is encapsulated as a
CommandResult
object which is passed back to theUi
.
Given below is the Sequence Diagram for interactions within the Logic
component for the execute("deleteModule mCode/CS2113")
API call.
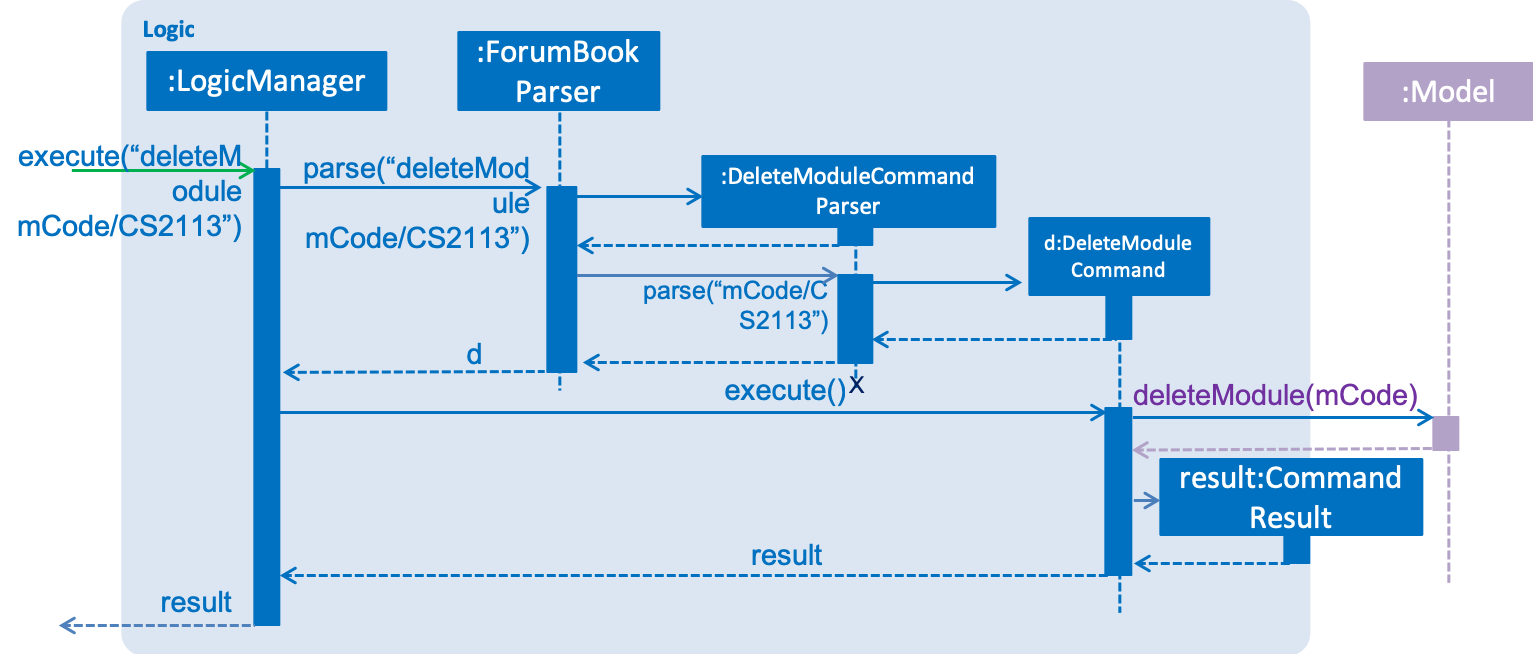
deleteModule mCode/CS2113
Command2.4. Storage component
The Storage
component,
-
can save
UserPref
objects in json format and read it back. -
can save the Forum Book data in encrypted json format and read it back.
2.5. Common classes
Classes used by multiple components are in the t13g2.forum.commons
package.
3. Implementation
This section describes some noteworthy details on how certain features are implemented.
3.1. CURD feature
3.1.1. Current Implementation
CURD refers to create
, update
, read
and delete
. This feature is implemented to achieve the basic functionalities of the Forum Book. It extends ForumBook
with various commands and supports the following operations:
-
createThread
: Create a new thread under a specific module that exists with comment. -
createComment
: Create a new comment under a specific thread that exists. -
updateThread
: Update an existing thread title created(unblocked) by its user. -
updateComment
: Update an existing comment content created(unblocked) by its user. -
listModule
: List out all the modules in the Forum Book. -
selectModule
: Select a specific module and list out all the threads under the module. -
selectThread
: Select a specific thread and list out all the comments under the thread. -
deleteThread
: Delete a specific thread. -
deleteComment
: Delete a specific comment.
Given below is an example usage scenario and how the CURD can be operated by user at each step.
Step 1. The user launches the application, executes createThread mCode/CS2113 tTitle/Exam Information cContent/What is the topic coverage for the final?
to create and add the thread into storage file. The createThread command calls UnitOfWork.commit()
, saving the modified forum book state into ForumBookStorage.
Step 2. The user executes createComment tId/123 cContent/This is a new comment content
to create and add the comment into storage file. The createComment command calls UnitOfWork.commit()
, saving the modified forum book state into ForumBookStorage.
Step 3. The user executes updateThread tId/123 tTitle/This is a new thread title
to update the title of the specific thread from storage file. The updateThread command calls UnitOfWork.getForumThreadRepository().getThread(threadId)
,forumThread.setTitle()
and lastly UnitOfWork.commit()
, saving the modified forum book state into ForumBookStorage.
Step 4. The user executes updateComment cId/123 cContent/This is a new comment content
to update the content of the specific comment from storage file. The updateComment command calls UnitOfWork.getCommentRepository().getComment(commentId)
,comment.setContent()
and lastly UnitOfWork.commit()
, saving the modified forum book state into ForumBookStorage.
Step 5. The user executes listModule
to retrieve all the existing modules from storage file. The listModule
command calls UnitOfWork.getModuleRepository().getAllModule()
which returns the module list to be shown in the panel.
Step 6. The user executes selectModule mCode/CS2113
to retrieve the module with module code CS2113 and its thread list from storage file. The selectModule
command calls UnitOfWork.getModuleRepository().getModuleByCode(moduleCode)
and UnitOfWork.getForumThreadRepository().getThreadsByModule(module)
to return the module and thread list to be shown in the panel.
Step 7. The user executes selectThread tId/123
to retrieve the thread with ID 123 and its comments from storage file. The selectModule
command calls UnitOfWork.getForumThreadRepository().getThread(threadId)
and UnitOfWork.getCommentRepository().getCommentsByThread(threadId)
to return the thread and comment list to be shown in the panel.
Step 8. The user executes deleteThread tId/123
to delete/remove the thread with ID 123 from storage file. The deleteThread command calls UnitOfWork.getForumThreadRepository().deleteThread(threadId)
and UnitOfWork.commit()
to save the modified forum book state.
Step 9. The user executes deleteComment cId/123
to delete/remove the comment with ID 123 from storage file. The deleteComment command calls UnitOfWork.getCommentRepository().deleteComment(commentId)
and UnitOfWork.commit()
to save the modified forum book state.
If the syntax of a command is wrong, the program will prompt the user to try again and show a suggested command format. |
3.2. Storage
3.2.1. Design Consideration
As the basis of the whole program, storage should be robust, efficient and stable at all the time. Data consistency and multi-thread access should be specially taken care of.
3.2.2. Overview
ForumBook’s storage consists of six(6) parts.
-
User
-
Stores user information like username, password, email etc.
-
-
Announcement
-
Stores announcement set by admin, multiple announcements can be saved in the storage.
-
-
Module
-
Stores module information like module name, code etc.
-
-
ForumThread
-
Stores threads posted by users
-
-
Comment
-
Stores comments under threads
-
-
RunningId
-
Used for generating auto incremented ID, each the above entity has a unique ID
-
These components are stored separately in six files for performance, see below for detailed process of loading and saving.
3.2.3. Storage Structure
To avoid unnecessary disk IO, each part of the storage is saved in a file located in forumData
directory.
All data manipulation must be done within an IUnitOfWork
where developer has to commit if the data
should be persistent on disk, or rollback if the exception is thrown somewhere during the transaction.
IStorage
defines interface to access file systems, this handles how our program actually get/read data.
By default, we have JsonFileStorage
which stores and reads data from local hard disk. More storage options
can be achieved by implementing this interface. eg: FtpStorage
.
IEncryptor
defines interface to encrypt application data, it should be injected to IStorage
providing a encryption/
decryption layer just before data writes and just after data reads. By default, JsonFileStorage
uses SimpleEncryptor
which is a simple 'OR' encryptor. A more secure encryption algorithm can be achieved by implementing IEncryptor
interface.
IForumBookStorage
defines interface to access data entities
I*Repository
defines interface to how we can manipulate different entities.
Each entity is managed by a repository, eg: IUserRepository
*Storage
is a thin wrapper for the List
containing data objects, it keeps track of whether the underling list
has been modified so that it can save some unnecessary disk IO if nothing changed when a commit()
is issued.
Data are saved in encrypted Json format, the encryption algorithm is provided by IEncryptor
RunningId
does not work as the other entities do, it has its own logic to make sure IDs are not repeated.
=== User management
3.2.4. Current Implementation
The user management is facilitated by various commands. It extends ForumBook
with a user management tool. Additionally, it implements the following operations:
-
AddUserCommand
— Add user to forum book. -
LoginCommand
: login to forum book. -
LogoutCommand
: logout from the forum book. -
UserDeleteCommand
: Self delete a user from the forum book.
Given below is an example usage scenario and how the user management behaves at each step.
-----------------------------------------------------------------------------------------------------------------------------------------------------
Given below is an example usage scenario and how the user management can be operated by user at each step.
Step 1. The user executes addUser uName/UserName uPass/password
to add a new user into forum userStorage file. The addUser command calls 'unitOfWork.getUserRepository().getUserByUsername(userToAdd.getUsername())' to check if the name entered by the user is available. If there is not duplicate, User can be registered. If the registration is successful, addUser command calls UnitOfWork.commit()
to save the modified forum book state into UserStorage.
Step 2. The user executes login uName/UserName uPass/password
to login to the forum. The login command calls 'unitOfWork.getUserRepository().authenticate(userName, userPassword)' to check if the login can be authorise. If success, login command calls Context.getInstance().setCurrentUser(exist)
to create an instance for the authorised user.
Step 3. The user executes logout
to exit the forum or to switch user, logout command calls 'EventsCenter.getInstance().post(new UserLoginEvent("", false, false))' to close the instance created by the user. Next/ another user is able to login after a successful logout.
ToDo: Step 4. The user executes `UserDeleteComment ` to remove himself/herself from the forum book. User data will be removed from the storage.
-----------------------------------------------------------------------------------------------------------------------------------------------------
=== Admin management
3.2.5. Current Implementation
The admin management is facilitated by various commands. It extends ForumBook
with a admin management tool. Additionally, it implements the following operations:
-
AnnounceCommand
— Posts new announcement. -
CheckAnnouncementCommand
: Checks for latest announcement. -
BlockUserFromCreatingCommand
: Blocks an existing user from posting either new threads or comments. -
SetAdminCommand
: Sets an existing user as admin or reverts an existing admin to user. -
CreateModuleCommand
: Creates a module by admin. -
UpdateModuleCommand
: Updates an existing module by admin. -
DeleteModuleCommand
: Deletes an existing module by admin. -
AdminUpdatePasswordCommand
: Updates an existing user’s password by admin. -
DeleteUserCommand
: Deletes an existing user by admin.
Given below is an example usage scenario and how the admin management behaves at each step.
Step 1. The admin launches the application, executes announce aTitle/Urgent! aContent/System maintenance from 3PM to 6PM.
to add the announcement to storage file. The announce
command calls UnitOfWork.getAnnouncementRepository.addAnnouncement(announcement)
and then UnitOfWork.commit()
, causing a modified forum book state to be saved into ForumBookStorage
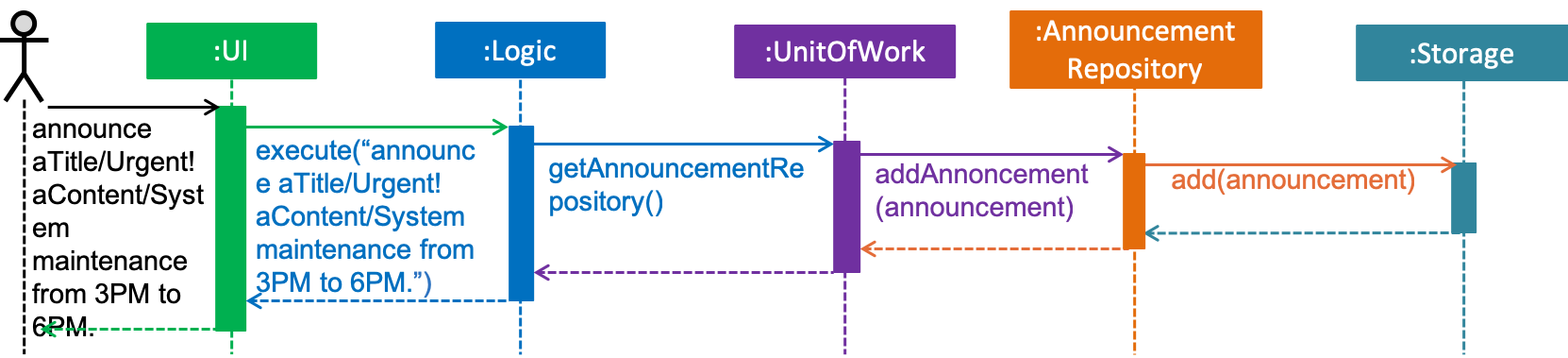
Step 2. The admin/user executes checkAnnounce
to check for the latest announcement in storage file. The checkAnnounce
calls the UnitOfWork.getAnnouncementRepository().getLatestAnnouncement()
which returns an announcement to be shown in the message dialog.
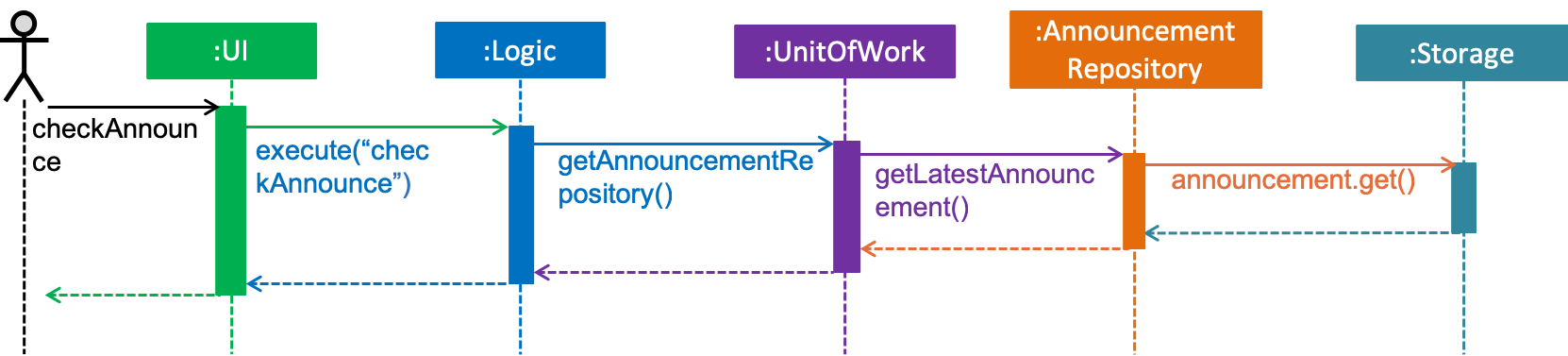
Step 3. The admin executes blockUser uName/john block/true
to block john from creating new threads or comments. The block
calls User.setIsBlock(true)
and then UnitOfWork.commit()
, causing another modified forum book state to be saved into ForumBookStorage
.
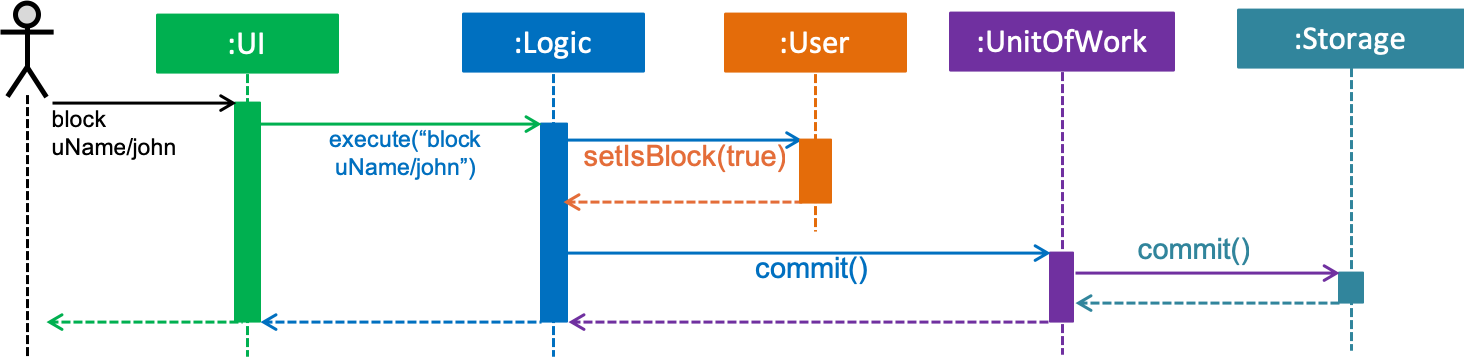
Step 4. The admin executes setAdmin uName/john set/true
to set a certain user as admin or to revert an admin to user. The setAdmin
calls the User.setAdmin(true)
and then UnitOfWork.commit()
, causing another modified forum book state to be saved into ForumBookStorage
.
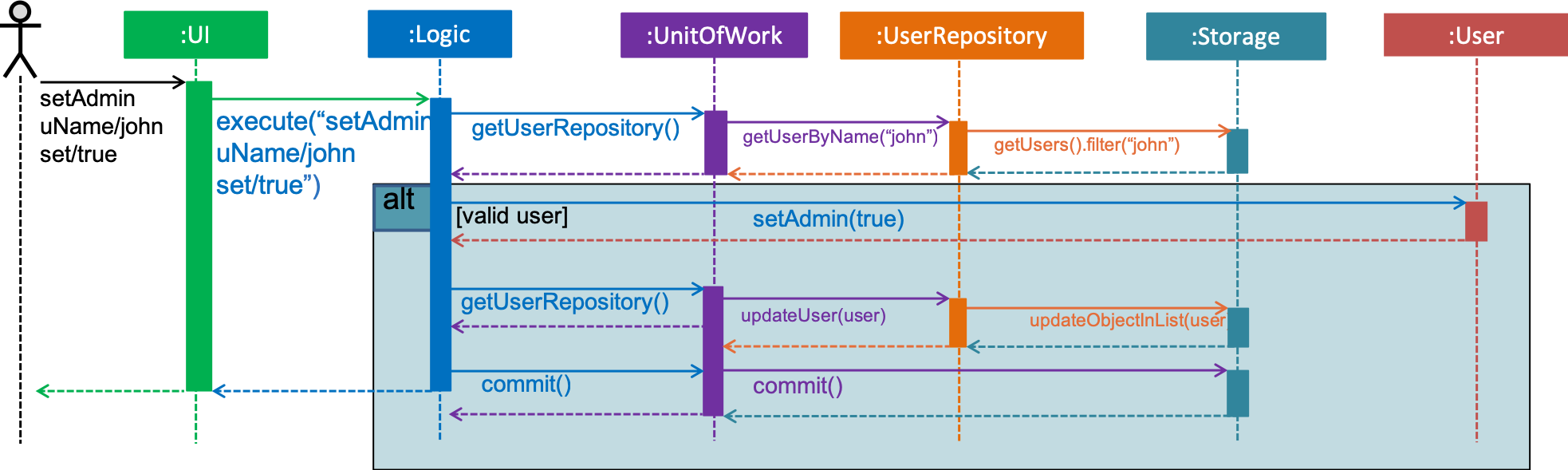
Step 5. The admin executes createModule mCode/CS2113 mTitle/Software Engineering and OOP
to create the specific module. The createModule
calls the UnitOfWork.getModuleRepository().addModule(module)
and then UnitOfWork.commit()
, causing another modified forum book state to be save into ForumBookStorage
.
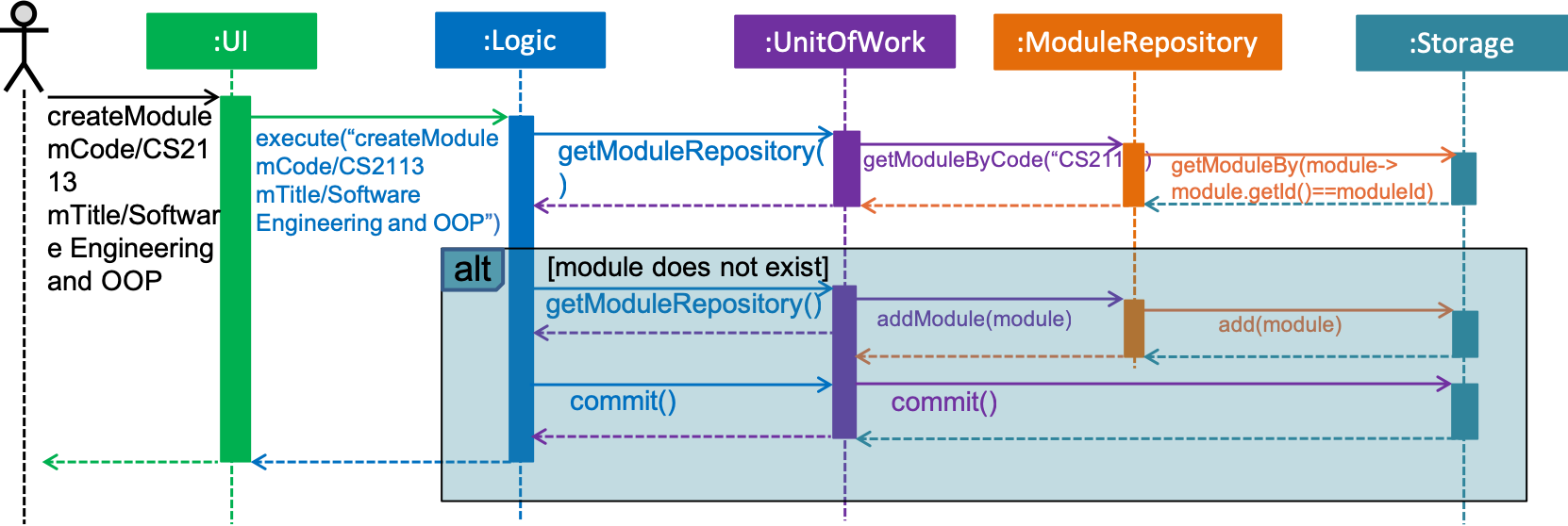
Step 6. The admin executes updateModule mId/3 mTitle/Software Eng and OOP
to update the specific module. The updateModule
calls the UnitOfWork.getModuleRepository().updateModule(module)
and then UnitOfWork.commit()
, causing another modified forum book state to be save into ForumBookStorage
.
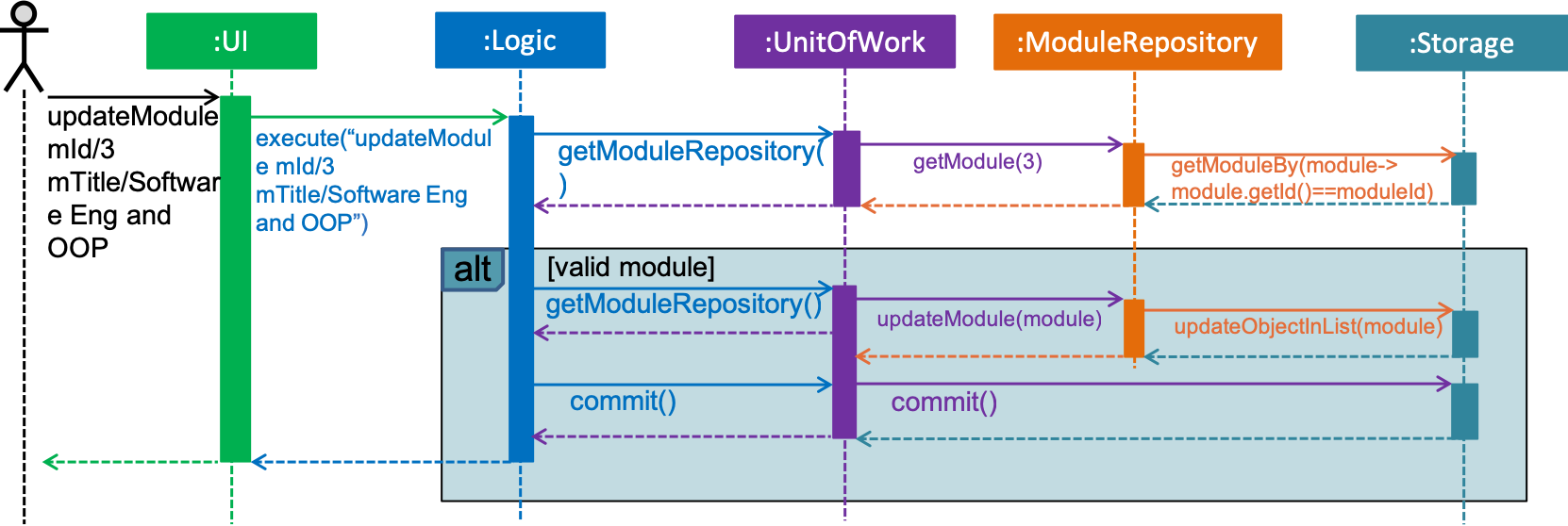
Step 7. The admin executes deleteModule mCode/CS2113
to delete specific module. The deleteModule
calls the UnitOfWork.getModuleRepository().removeModule(module);
and then UnitOfWork.commit()
, causing another modified forum book state to be save into ForumBookStorage
.
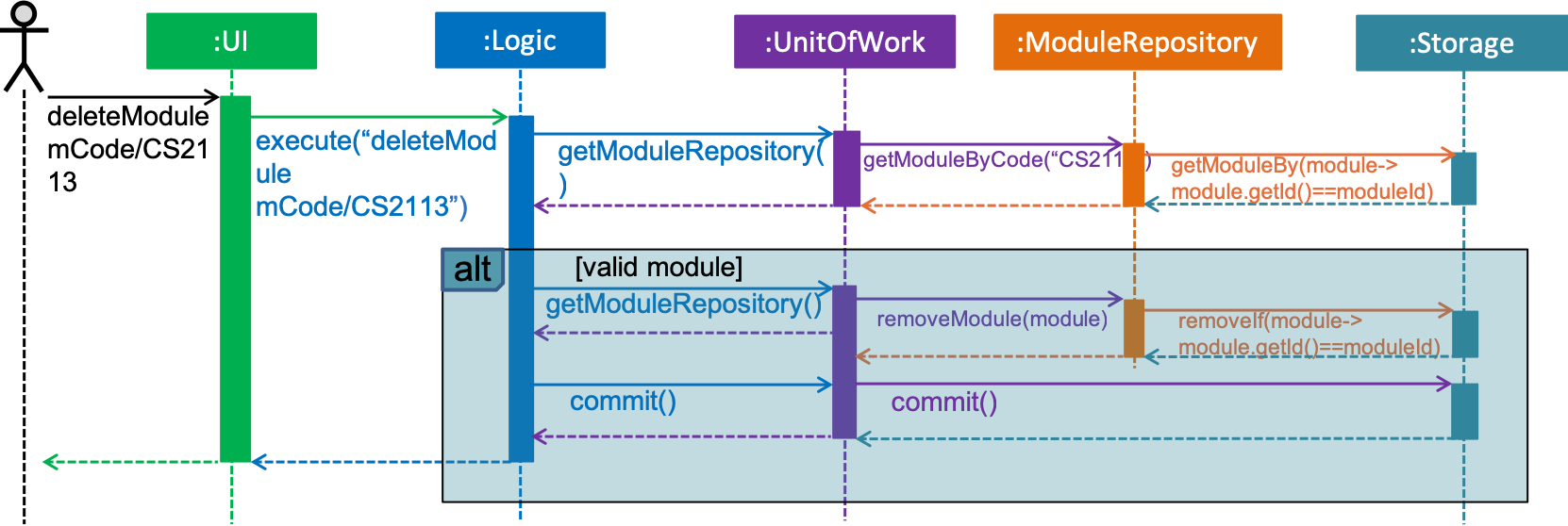
Step 8. The admin executes updatePass uName/john uPass/098
to update the user john’s password. The updatePass
calls the UnitOfWork.getUserRepository().updateUser(user)
and then UnitOfWork.commit()
, causing another modified forum book state to be save into ForumBookStorage
.
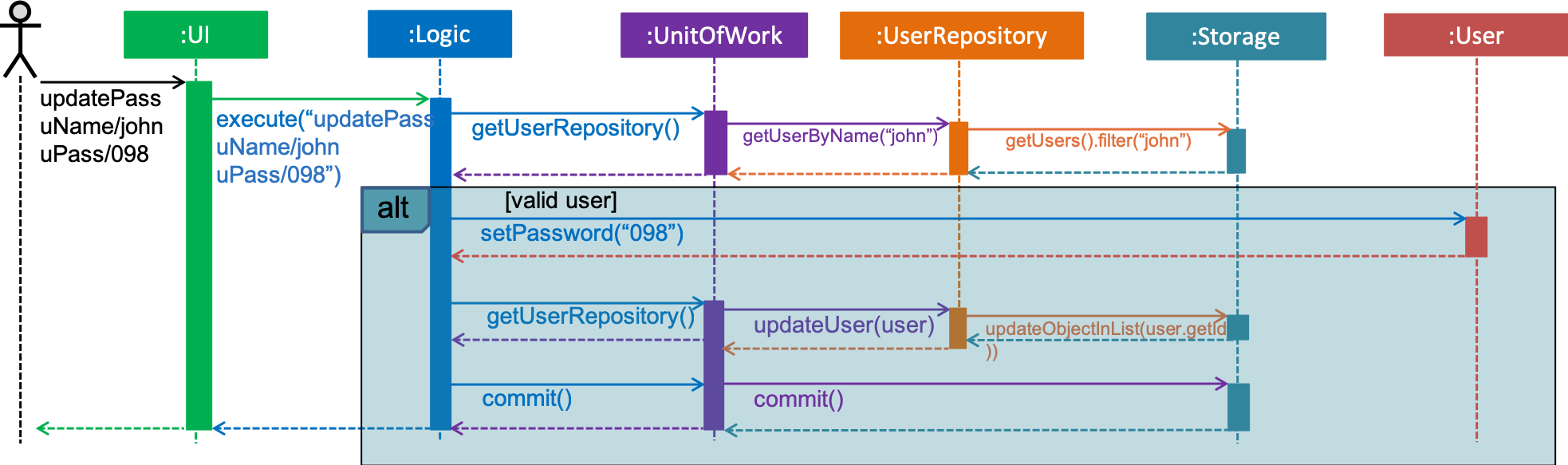
Step 9. The admin executes deleteUser uName/john
to delete the user john. The deleteUser
calls the ` UnitOfWork.getUserRepository().deleteUser(userToDelete)` and then UnitOfWork.commit()
, causing another modified forum book state to be save into ForumBookStorage
.
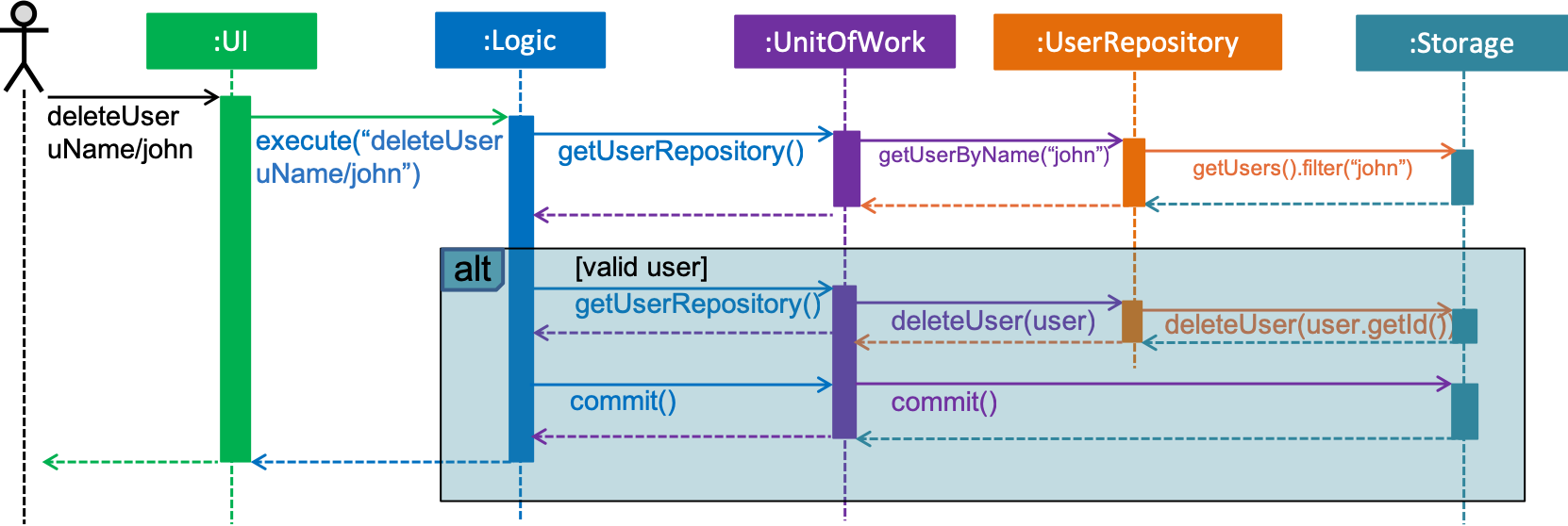
If the syntax of a command is wrong, the program will prompt the user to try again and show a suggested command format. |
3.3. Data Encryption
Data encryption is done in a transparent layer.
All data in this application is encrypted.
A very simple, naive, and insecure encryption is implemented in SimpleEncryptor
, this is only for demoing the ability of
encrypting data in this application. However, a more secure algorithm can be easily added into the app by
implementing the IEncryptor
interface and provide it into IStorage
.
3.4. Logging
We are using java.util.logging
package for logging. The LogsCenter
class is used to manage the logging levels and logging destinations.
-
The logging level can be controlled using the
logLevel
setting in the configuration file (See Section 3.5, “Configuration”) -
The
Logger
for a class can be obtained usingLogsCenter.getLogger(Class)
which will log messages according to the specified logging level -
Currently log messages are output through:
Console
and to a.log
file.
Logging Levels
-
SEVERE
: Critical problem detected which may possibly cause the termination of the application -
WARNING
: Can continue, but with caution -
INFO
: Information showing the noteworthy actions by the App -
FINE
: Details that is not usually noteworthy but may be useful in debugging e.g. print the actual list instead of just its size
3.5. Configuration
Certain properties of the application can be controlled (e.g App name, logging level) through the configuration file (default: config.json
).
4. Documentation
We use asciidoc for writing documentation.
We chose asciidoc over Markdown because asciidoc, although a bit more complex than Markdown, provides more flexibility in formatting. |
4.1. Editing Documentation
See UsingGradle.adoc to learn how to render .adoc
files locally to preview the end result of your edits.
Alternatively, you can download the AsciiDoc plugin for IntelliJ, which allows you to preview the changes you have made to your .adoc
files in real-time.
4.2. Publishing Documentation
See UsingTravis.adoc to learn how to deploy GitHub Pages using Travis.
4.3. Converting Documentation to PDF format
We use Google Chrome for converting documentation to PDF format, as Chrome’s PDF engine preserves hyperlinks used in webpages.
Here are the steps to convert the project documentation files to PDF format.
-
Follow the instructions in UsingGradle.adoc to convert the AsciiDoc files in the
docs/
directory to HTML format. -
Go to your generated HTML files in the
build/docs
folder, right click on them and selectOpen with
→Google Chrome
. -
Within Chrome, click on the
Print
option in Chrome’s menu. -
Set the destination to
Save as PDF
, then clickSave
to save a copy of the file in PDF format. For best results, use the settings indicated in the screenshot below.
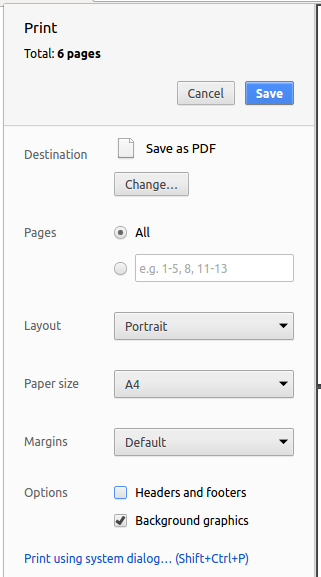
4.4. Site-wide Documentation Settings
The build.gradle
file specifies some project-specific asciidoc attributes which affects how all documentation files within this project are rendered.
Attributes left unset in the build.gradle file will use their default value, if any.
|
Attribute name | Description | Default value |
---|---|---|
|
The name of the website. If set, the name will be displayed near the top of the page. |
not set |
|
URL to the site’s repository on GitHub. Setting this will add a "View on GitHub" link in the navigation bar. |
not set |
4.5. Per-file Documentation Settings
Each .adoc
file may also specify some file-specific asciidoc attributes which affects how the file is rendered.
Asciidoctor’s built-in attributes may be specified and used as well.
Attributes left unset in .adoc files will use their default value, if any.
|
Attribute name | Description | Default value |
---|---|---|
|
Site section that the document belongs to.
This will cause the associated item in the navigation bar to be highlighted.
One of: |
not set |
|
Set this attribute to remove the site navigation bar. |
not set |
4.6. Site Template
The files in docs/stylesheets
are the CSS stylesheets of the site.
You can modify them to change some properties of the site’s design.
The files in docs/templates
controls the rendering of .adoc
files into HTML5.
These template files are written in a mixture of Ruby and Slim.
Modifying the template files in |
5. Testing
5.1. Running Tests
There are three ways to run tests.
The most reliable way to run tests is the 3rd one. The first two methods might fail some GUI tests due to platform/resolution-specific idiosyncrasies. |
Method 1: Using IntelliJ JUnit test runner
-
To run all tests, right-click on the
src/test/java
folder and chooseRun 'All Tests'
-
To run a subset of tests, you can right-click on a test package, test class, or a test and choose
Run 'ABC'
Method 2: Using Gradle
-
Open a console and run the command
gradlew clean allTests
(Mac/Linux:./gradlew clean allTests
)
See UsingGradle.adoc for more info on how to run tests using Gradle. |
Method 3: Using Gradle (headless)
Thanks to the TestFX library we use, our GUI tests can be run in the headless mode. In the headless mode, GUI tests do not show up on the screen. That means the developer can do other things on the Computer while the tests are running.
To run tests in headless mode, open a console and run the command gradlew clean headless allTests
(Mac/Linux: ./gradlew clean headless allTests
)
5.2. Types of tests
We have two types of tests:
-
GUI Tests - These are tests involving the GUI. They include,
-
System Tests that test the entire App by simulating user actions on the GUI. These are in the
systemtests
package. -
Unit tests that test the individual components. These are in
t13g2.forum.ui
package.
-
-
Non-GUI Tests - These are tests not involving the GUI. They include,
-
Unit tests targeting the lowest level methods/classes.
e.g.t13g2.forum.commons.StringUtilTest
-
Integration tests that are checking the integration of multiple code units (those code units are assumed to be working).
e.g.StorageManagerTest
-
Hybrids of unit and integration tests. These test are checking multiple code units as well as how the are connected together.
e.g.LogicManagerTest
-
5.3. Troubleshooting Testing
Problem: HelpWindowTest
fails with a NullPointerException
.
-
Reason: One of its dependencies,
HelpWindow.html
insrc/main/resources/docs
is missing. -
Solution: Execute Gradle task
processResources
.
6. Dev Ops
6.1. Build Automation
See UsingGradle.adoc to learn how to use Gradle for build automation.
6.2. Continuous Integration
We use Travis CI and AppVeyor to perform Continuous Integration on our projects. See UsingTravis.adoc and UsingAppVeyor.adoc for more details.
6.3. Coverage Reporting
We use Coveralls to track the code coverage of our projects. See UsingCoveralls.adoc for more details.
6.4. Documentation Previews
When a pull request has changes to asciidoc files, you can use Netlify to see a preview of how the HTML version of those asciidoc files will look like when the pull request is merged. See UsingNetlify.adoc for more details.
6.5. Making a Release
Here are the steps to create a new release.
-
Update the version number in
MainApp.java
. -
Generate a JAR file using Gradle.
-
Tag the repo with the version number. e.g.
v0.1
-
Create a new release using GitHub and upload the JAR file you created.
6.6. Managing Dependencies
A project often depends on third-party libraries. For example, Address Book depends on the Jackson library for XML parsing. Managing these dependencies can be automated using Gradle. For example, Gradle can download the dependencies automatically, which is better than these alternatives.
a. Include those libraries in the repo (this bloats the repo size)
b. Require developers to download those libraries manually (this creates extra work for developers)
Appendix A: Product Scope
Target user profile:
-
has a need to share and read comments related to modules
-
prefer desktop apps over other types
-
can type fast
-
prefers typing over mouse input
-
is reasonably comfortable using CLI apps
Value proposition: Read a share comments faster than a typical mouse/GUI driven app
Appendix B: User Stories
Priorities: High (must have) - * * *
, Medium (nice to have) - * *
, Low (unlikely to have) - *
Priority | As a … | I want to … | So that I can… |
---|---|---|---|
|
new user |
see usage instructions |
refer to instructions when I forget how to use the App |
|
student who is taking a certain module in current semester |
create a new forumThread |
share the exam information with others |
|
student who is going to take a certain module in future semesters |
select that module and corresponding threads and comments under the module |
learn more about the module in advance |
|
student |
list all existing modules |
check what modules are available to interact with other students |
|
forum thread owner |
update my thread title |
correct my typing error in this thread title |
|
forum thread owner |
delete my thread |
close down the thread for further discussion under this thread |
|
comment owner |
update my comment content |
correct my typing error or my thoughts in this comment |
|
comment owner |
delete my comment |
|
|
ForumBook admin |
announce |
show some important information to users |
|
User |
add myself as a user |
register and use the forum book |
|
User |
login and logout |
share my comments in the forum book and exit when i want to |
|
User |
delete myself from being a user |
|
|
ForumBook admin |
block users |
prevent users from creating and updating threads and comments |
|
ForumBook admin |
change user password |
help the user to reset the password once they forget their password |
|
ForumBook admin |
set admin |
have more admins to manage the ForumBook better |
{More to be added}
Appendix C: Use Cases
(For all use cases below, the System is the ForumBook
and the Actor is the user
, unless specified otherwise)
Use case: List all threads under CS2113
MSS
-
User requests to list all modules
-
ForumBook shows a list of modules
-
User requests to list all threads under module CS2113
-
ForumBook shows a list of threads under module CS2113
Use case ends.
Extensions
-
2a. The list is empty.
Use case ends.
-
3a. The given module code is invalid.
-
3a1. ForumBook shows an error message.
Use case ends.
-
C.1. Use case: Create new thread under CS2113
MSS
-
User requests to list all modules
-
ForumBook shows a list of modules
-
User requests to create new thread under module CS2113
-
ForumBook adds the new thread and shows a success message
Use case ends.
Extensions
-
2a. The list is empty.
-
2a1. ForumBook shows empty list of module
Use case ends.
-
-
3a. The given module code is invalid.
-
3a1. ForumBook shows an error message.
Use case ends.
-
C.2. Use case: Create new comment
MSS
-
User requests to list all modules
-
ForumBook shows a list of modules
-
User requests to create new comment
-
ForumBook adds the new comment and shows a success message
Use case ends.
Extensions
-
2a. The list is empty.
-
2a1. ForumBook shows empty list of module
Use case ends.
-
-
3a. The given thread id is invalid.
-
3a1. ForumBook shows an error message.
Use case ends.
-
C.3. Use case: Delete thread and comment
MSS
-
User requests to delete a thread
-
ForumBook deletes the thread and shows success message
-
User requests to delete a comment
-
ForumBook deletes the comment and shows success message
Use case ends.
Extensions
-
2a. current user is not the thread owner.
-
2a1. ForumBook shows error message
Use case ends.
-
-
3a. current user is not the comment owner.
-
3a1. ForumBook shows an error message.
Use case ends.
-
C.4. Use case: Update thread and comment
MSS
-
User requests to update a thread
-
ForumBook updates the thread and shows success message
-
User requests to update a comment
-
ForumBook updates the comment and shows success message
Use case ends.
Extensions
-
2a. current user is not the thread owner.
-
2a1. ForumBook shows error message
Use case ends.
-
-
3a. current user is not the comment owner.
-
3a1. ForumBook shows an error message.
Use case ends.
-
{More to be added}
Appendix D: Non Functional Requirements
-
Should work on any mainstream OS as long as it has Java
9
or higher installed. -
Should be able to hold up to 1000 threads with comments without a noticeable sluggishness in performance for typical usage.
-
A user with above average typing speed for regular English text (i.e. not code, not system admin commands) should be able to accomplish most of the tasks faster using commands than using the mouse.
Appendix F: Instructions for Manual Testing
Given below are instructions to test the app manually.
These instructions only provide a starting point for testers to work on; testers are expected to do more exploratory testing. |
F.1. Launch and Shutdown
-
Initial launch
-
Download the jar file and copy into an empty folder
-
Double-click the jar file
Expected: Shows the GUI with a set of sample contacts. The window size may not be optimum.
-
-
Getting sample data file
-
Download [sample forumData zip folder] and unzip
-
Replace the default data folder generated by the application with the forumData folder you have downloaded.
-
On relaunching the application, new data should be loaded.
-
-
Saving window preferences
-
Resize the window to an optimum size. Move the window to a different location. Close the window.
-
Re-launch the app by double-clicking the jar file.
Expected: The most recent window size and location is retained.
-
F.2. Registering and logging in
-
Registering a new user
-
Prerequisites: username must not be registered before.
-
Test case:
addUser uName/john uPass/123
Expected: the userjohn
will be added to the UserStorage if there is no duplicate in the UserStorage.
-
-
Loggin in
-
Test case:
login uName/abcd uPass/123
Expected: Log in with a random username and password that has not been registered. An error message will be shown in the result display panel. -
Test case:
login uName/john uPass/234
Expected: Log in with a registered username and a wrong password. An error message will be shown in the result display panel. -
Test case:
login uName/john uPass/123
Expected: Log in with a registered username and correct password, a success message will be shown in the result display panel.
-
F.3. Announcing new announcement
-
Announcing a new announcement by admin.
-
Prerequisites: Current user must login as an admin.
-
Test case:
announce aTitle/Urgent! aContent/System Maintenance from 4pm to 5pm.
Expected: Announcement will show up in a pop up window as well as the result display panel. -
Test case:
announce aTitle/ aContent/
Expected: No announcement will be posted. Error details shown in the result display panel.
-
F.4. Checking new announcement
-
Checking for latest announcement.
-
Prerequisites: Users have to login in order to do
checkAnnounce
-
Test case:
checkAnnounce
Expected: The latest announcement will show up in a pop up window and in the result display panel. However, if the latest announcement is null, an error message "There is no announcement at the moment" will be shown in the result display panel.
-
F.5. Blocking user from posting
-
Blocking a given user by admin.
-
Prerequisites: Current user must login as an admin. The given user should not be an admin and should be in the UserStorage.
-
Test case:
blockUser uName/john block/true
Expected: The given user will be blocked and a success message will be shown in the result display panel. -
Test case:
blockUser uName/abcd block/true
Expected: Since the given user has not been added to the UserStorage, an error message will be shown in the result display panel.
-
F.6. Setting a user as admin or setting an admin as user
. Setting a user as admin or revert an admin to a user.
-
Prerequisites: Current user must login as an admin. Admin is unable to set/revert himself/herself to admin/user. The given user must not been blocked and must be in UserStorage.
-
Test case:
setAdmin uName/john set/true
Expected: The given user will be set to an admin and the success message will be shown in the result display panel. -
Test case:
setAdmin uName/abcd set/true
Expected: Since the given user has not been added to the UserStorage, an error message will be shown in the result display panel.
F.7. Creating a module
-
Creating a module by admin.
-
Prerequisites: Current user must login as an admin. The module must not be in the ModuleStorage.
-
Test case:
createModule mCode/CS2113 mContent/Software Engineering and OOP
Expected: A new module will be added to the ModuleStorage, and a success message will be shown in the result display panel. -
Test case:
createModule mCode/ mTitle/
Expected: No module will be added. Error details shown in the result display panel.
-
F.8. Creating a thread
-
Creating a thread under certain module by user or admin.
-
Prerequisites: User must login to proceed. User should not be blocked by admin.
-
Test case:
createThread mCode/CS2113 tTitle/Exam Information cContent/All the best for the final guys
Expected: A new thread will be added to the ForumThreadStorage, and a success message will be shown in the result display panel. -
Test case:
createThread mCode/ tTitle/ cContent/
Expected: No module will be added. Error details shown in the result display panel.
-
F.9. Creating a comment
-
Creating a comment under certain forum thread by user or admin.
-
Prerequisites: User must login to proceed. User should not be blocked by admin.
-
Test case:
createComment tId/1 cContent/This is a new comment
Expected: A new thread will be added to the CommentStorage, and a success message will be shown in the result display panel. -
Test case:
createComment tId/ cContent/
Expected: No comment will be added. Error details shown in the result display panel.
-
F.10. Updating a thread title
-
Updating the title of a forum thread by thread creator or admin.
-
Prerequisites: User must login to proceed. User is the owner/creator of the forum thread.
-
Test case: (If thread ID 123 is under current module) +
updateThread tId/123 tTitle/This is a new title
Expected: The new thread title will be updated in the ForumThreadStorage, and a success message will be shown in the result display panel. -
Test case: (If thread ID 123 does not exist in the forum book) +
updateThread tId/123 tTitle/This is a new title
Expected: No thread title will be updated. Error details of invalid thread id will be shown in the result display panel. -
Test case: (If thread ID 123 is not under current module) +
updateThread tId/123 tTitle/This is a new title
Expected: No thread title will be updated. Error details of entity is not under scope will be shown in the result display panel. -
Test case:
updateThread tId/ tTitle/
Expected: No thread title will be updated. Error details shown in the result display panel.
-
F.11. Updating a comment content
-
Updating the content of a comment by comment creator or admin.
-
Prerequisites: User must login to proceed. User is the owner/creator of the comment.
-
Test case: (If comment ID 123 is under current forum thread) +
updateThread cId/123 cContent/This is a new content
Expected: The new comment content will be updated in the CommentStorage, and a success message will be shown in the result display panel. -
Test case: (If comment ID 123 does not exist in the forum book) +
updateThread cId/123 cContent/This is a new content
Expected: No comment content will be updated. Error details of invalid comment id will be shown in the result display panel. -
Test case: (If comment ID 123 is not under current forum thread) +
updateThread cId/123 cContent/This is a new content
Expected: No comment content will be updated. Error details of entity is not under scope will be shown in the result display panel. -
Test case:
updateThread cId/ cContent/
Expected: No comment content will be updated. Error details shown in the result display panel.
-
F.12. Deleting a thread
-
Deleting a forum thread by thread creator or admin.
-
Prerequisites: User must login to proceed. User is the owner/creator of the forum thread.
-
Test case: (If thread ID 123 is under current module) +
deleteThread tId/123
Expected: The new thread title will be deleted in the ForumThreadStorage, and a success message will be shown in the result display panel. -
Test case: (If thread ID 123 does not exist in the forum book) +
deleteThread tId/123
Expected: No thread title will be deleted. Error details of invalid thread id will be shown in the result display panel. -
Test case: (If thread ID 123 is not under current module) +
deleteThread tId/123
Expected: No thread title will be deleted. Error details of entity is not under scope will be shown in the result display panel. -
Test case:
deleteThread tId/
Expected: No thread title will be deleted. Error details shown in the result display panel.
-
F.13. Deleting a comment
-
Deleting a comment by comment creator or admin.
-
Prerequisites: User must login to proceed. User is the owner/creator of the comment.
-
Test case: (If comment ID 123 is under current forum thread) +
deleteComment cId/123
Expected: The new comment content will be deleted in the CommentStorage, and a success message will be shown in the result display panel. -
Test case: (If comment ID 123 does not exist in the forum book) +
deleteComment cId/123
Expected: No comment content will be deleted. Error details of invalid comment id will be shown in the result display panel. -
Test case: (If comment ID 123 is not under current forum thread) +
deleteComment cId/123
Expected: No comment content will be deleted. Error details of entity is not under scope will be shown in the result display panel. -
Test case:
deleteComment cId/
Expected: No comment content will be deleted. Error details shown in the result display panel.
-
F.14. Listing all modules
-
Listing all the modules in the forun book by user or admin.
-
Prerequisites: User must login to proceed.
-
Test case:
listModule
Expected: A list of modules in the ModuleStorage will be shown in the result display panel.
-
F.15. Selecting a module to list all threads
-
Listing all the forum threads under certain module in the forun book by user or admin.
-
Prerequisites: User must login to proceed. The module code entered by user should exist in the ModuleStorage.
-
Test case:
selectModule mCode/CS2113
Expected: A list of forum threads under module CS2113 in the ForumThreadStorage will be shown in the result display panel. -
Test case:
selectModule mCode/
Expected: No forum thread will be listed out. Error details shown in the result display panel.
-
F.16. Selecting a thread to list all comments
-
Listing all the comments under certain forum thread in the forun book by user or admin.
-
Prerequisites: User must login to proceed. The thread Id entered by user should exist in the ForumThreadStorage.
-
Test case:
selectThread tId/123
Expected: A list of comments under thread ID 123 in the ForumThreadStorage will be shown in the result display panel. -
Test case:
selectModule tId/
Expected: No comment will be listed out. Error details shown in the result display panel.
-
F.17. Updating a module
-
Updating a module by admin.
-
Prerequisites: Current user must login as an admin. The module must be in ModuleStorage.
-
Test case:
updateModule mId/3 mCode/CS1223
Expected: The moduleCS2113
will be updated to take the module codeCS1223
, and an success message will be shown in the result display panel. -
Test case:
updateModule mCode/CS1223
Expected: Since the module id was not specified, The module will not be updated. This command should raise an error and show in the result display panel.
-
F.18. Deleting a module
-
Deleting a module by admin.
-
Prerequisites: Current user must login as an admin. The module must be in ModuleStorage.
-
Test case:
deleteModule mCode/CS2113
Expected: The moduleCS2113
will be deleted from the ModuleStorage, and an success message will be shown in the result display panel. -
Test case:
deleteModule mCode/CS2113
Expected: Since the moduleCS2113
has been deleted. This command should raise an error and show in the result display panel.
-
F.19. Updating password
-
Updating a given user’s password by admin.
-
Prerequisites: Current user must login as an admin. The given user must be in UserStorage.
-
Test case:
updatePass uName/john uPass/456
Expected: The userjohn’s password will be updated to `456
-
Test case:
updatePass uName/abcd uPass/123
Expected: Since the given userabcd
has not been added to the UserStorage, an error message will be shown in the result display panel.
-
F.20. Deleting a user(Admin)
-
Deleting a given user by admin.
-
Prerequisites: Current user must login as an admin. The given user must be in the UserStorage.
-
Test case:
deleteUser uName/john
Expected: The userjohn
will be deleted from the UserStorage and a success message will be shown in the result display panel. -
Test case:
deleteUser uName/abcd
Expected: Since the given userabcd
does not exist in the UserStorage, an error message will be shown in the result display panel.
-
F.21. Deleting a user(User)
-
Self Deleting a user.
-
Prerequisites: User who is interested in removing himself from the forum must login to his account. The given user must be in the UserStorage.
-
Test case:
deleteMe
-user logged in Expected Success: The current user will be deleted from the UserStorage and a success message will be shown in the result display panel. -
Test case:
deleteMe
-user not logged in Expected Fail: Since the given user is not logged in, an error message will be shown in the result display panel.
-